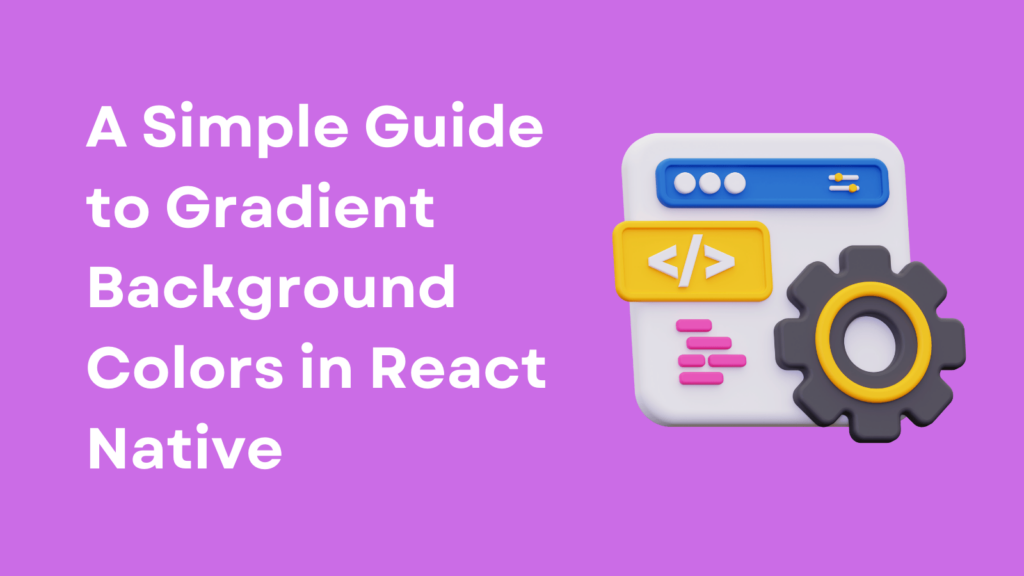
Adding gradient background colors can give your React Native app a modern and visually appealing look. Gradients are smooth transitions between two or more colors, and they can be used to enhance the design of your app’s UI. In this guide, we’ll show you how to create gradient backgrounds in React Native using simple steps and code examples.
What is a Gradient Background?
A gradient background is a visual effect where colors blend smoothly from one to another. It can be a linear gradient (colors blend along a straight line) or a radial gradient (colors blend from a central point outward). Gradients are often used to make backgrounds more dynamic and visually interesting.
How to Implement Gradient Background Colors in React Native
To use gradient backgrounds in React Native, you need to install a library called react-native-linear-gradient
. This library provides a component for creating linear gradients.
1. Install react-native-linear-gradient
First, add the react-native-linear-gradient
package to your project. Open your terminal and run:
npm install react-native-linear-gradient
Then, link the package (for React Native CLI projects):
npx pod-install
2. Using Linear Gradient
Once you’ve installed the package, you can start using the LinearGradient
component. Here’s a basic example:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
import LinearGradient from 'react-native-linear-gradient';
const App = () => {
return (
<LinearGradient
colors={['#FF5733', '#FFBD33']}
style={styles.gradient}
>
<View style={styles.container}>
<Text style={styles.text}>Gradient Background!</Text>
</View>
</LinearGradient>
);
};
const styles = StyleSheet.create({
gradient: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
container: {
backgroundColor: 'transparent',
},
text: {
fontSize: 24,
color: '#fff',
},
});
export default App;
3. Customize Your Gradient
You can customize your gradient background by adjusting the following properties:
colors
: An array of color codes that define the gradient. For example,['#FF5733', '#FFBD33']
creates a gradient from orange to yellow.start
: Defines the starting point of the gradient. For instance,{ x: 0, y: 0 }
means the gradient starts at the top-left corner.end
: Defines the ending point of the gradient. For example,{ x: 1, y: 1 }
means the gradient ends at the bottom-right corner.
Here’s an example with a customized gradient:
<LinearGradient
colors={['#FF5733', '#C70039']}
start={{ x: 0, y: 0 }}
end={{ x: 1, y: 1 }}
style={styles.gradient}
>
<View style={styles.container}>
<Text style={styles.text}>Custom Gradient Background!</Text>
</View>
</LinearGradient>
4. Radial Gradients
react-native-linear-gradient
supports only linear gradients. For radial gradients, you can use the react-native-svg
library to create complex shapes and gradients.
Here’s a basic example of a radial gradient with react-native-svg
:
npm install react-native-svg
import React from 'react';
import { View } from 'react-native';
import Svg, { Defs, RadialGradient, Stop, Rect } from 'react-native-svg';
const RadialGradientExample = () => {
return (
<View style={{ flex: 1 }}>
<Svg height="100%" width="100%" viewBox="0 0 100 100">
<Defs>
<RadialGradient id="grad1" cx="50%" cy="50%" r="50%" fx="50%" fy="50%">
<Stop offset="0%" style={{ stopColor: '#FF5733', stopOpacity: 1 }} />
<Stop offset="100%" style={{ stopColor: '#C70039', stopOpacity: 1 }} />
</RadialGradient>
</Defs>
<Rect width="100%" height="100%" fill="url(#grad1)" />
</Svg>
</View>
);
};
export default RadialGradientExample;
Best Practices for Gradient Backgrounds
- Keep It Subtle: Avoid overly bright or complex gradients that can distract from the content.
- Ensure Readability: Make sure text and other elements are readable against the gradient background.
- Test on Different Devices: Gradients can look different on various screen types and sizes, so test your design across devices.
Conclusion
Gradient backgrounds can add a visually appealing touch to your React Native app. By using the react-native-linear-gradient
library, you can easily create linear gradients, and with react-native-svg
, you can explore more complex radial gradients. Customize your gradients to fit your app’s design and create a stunning user experience.
Happy coding! 🎨
Leave a Reply