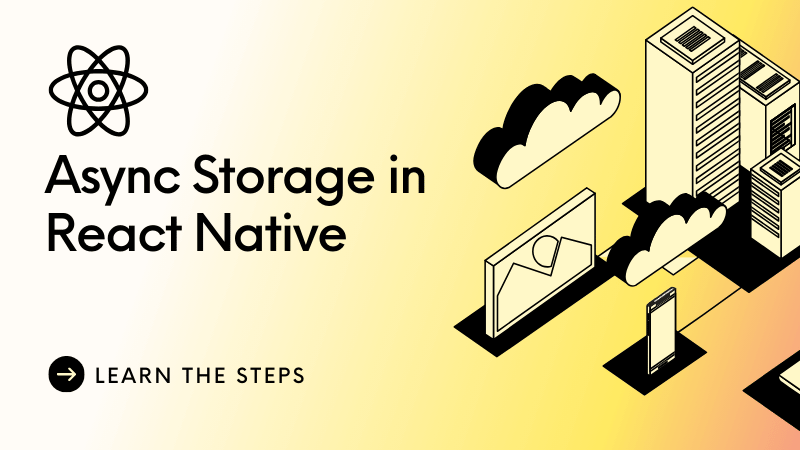
React Native provides an easy way to store data on a device using Async Storage. If you’re building an app that needs to remember user settings, login sessions, or any other small amount of data between app launches, Async Storage is a great tool to use.
In this blog, I’ll walk you through what Async Storage is, how to set it up, and how to use it with simple code examples. I promise to keep things simple and easy to follow!
What is Async Storage?
Async Storage is a simple key value storage system that allows you to store data on the device. It’s useful for saving things like:
- User preferences (e.g., dark mode, language settings)
- Tokens (e.g., authentication tokens)
- Simple app data (e.g., last opened screen)
It’s not meant for large datasets or complex databases. For that, you might want to look into databases like SQLite or Realm.
How to Install Async Storage
First, you need to install the package. Open your terminal and run the following command in your React Native project:
npm install @react-native-async-storage/async-storage
After it’s installed, link the package with your project:
npx pod-install
You’re all set!
How to Use Async Storage
Now let’s look at some basic examples of how to use Async Storage to store and retrieve data in your React Native app.
1. Saving Data with Async Storage
Let’s say you want to save the user’s name when they log in. Here’s how you would do it:
import AsyncStorage from '@react-native-async-storage/async-storage';
const saveName = async (name) => {
try {
await AsyncStorage.setItem('userName', name);
console.log('Name saved!');
} catch (error) {
console.error('Failed to save the name.', error);
}
};
In this example, we use the setItem
function to store a value (the user’s name) with a key ('userName'
). The key is like a label, and it’s how we’ll get the data back later.
2. Retrieving Data from Async Storage
Now that we’ve saved the user’s name, let’s retrieve it when the app starts:
import AsyncStorage from '@react-native-async-storage/async-storage';
const getName = async () => {
try {
const name = await AsyncStorage.getItem('userName');
if (name !== null) {
console.log('User name is:', name);
}
} catch (error) {
console.error('Failed to fetch the name.', error);
}
};
Here, we use the getItem
function and pass the key ('userName'
) to retrieve the stored value.
3. Removing Data
You might also want to remove data, for example, when a user logs out:
import AsyncStorage from '@react-native-async-storage/async-storage';
const removeName = async () => {
try {
await AsyncStorage.removeItem('userName');
console.log('Name removed!');
} catch (error) {
console.error('Failed to remove the name.', error);
}
};
With removeItem
, the data stored with the key 'userName'
is deleted from storage.
4. Clearing All Storage
If you want to clear everything stored in Async Storage, use the clear
method:
import AsyncStorage from '@react-native-async-storage/async-storage';
const clearAllData = async () => {
try {
await AsyncStorage.clear();
console.log('All data cleared!');
} catch (error) {
console.error('Failed to clear storage.', error);
}
};
This will remove all data saved in Async Storage, so use it carefully!
Best Practices for Async Storage
- Keep it simple: Async Storage is best for small pieces of data. If you need to store larger amounts of data, consider using a database.
- Error Handling: Always include
try/catch
blocks to handle errors, like storage being full or unexpected issues. - Stringify Complex Data: Async Storage only stores strings. If you want to store objects or arrays, use
JSON.stringify()
before saving, andJSON.parse()
when retrieving.
Example:
const saveUser = async (user) => {
try {
const userString = JSON.stringify(user);
await AsyncStorage.setItem('userDetails', userString);
console.log('User saved!');
} catch (error) {
console.error('Failed to save user data.', error);
}
};
const getUser = async () => {
try {
const userString = await AsyncStorage.getItem('userDetails');
if (userString !== null) {
const user = JSON.parse(userString);
console.log('User data:', user);
}
} catch (error) {
console.error('Failed to fetch user data.', error);
}
};
In this example, we convert an object to a string using JSON.stringify
before saving it and use JSON.parse
to convert it back to an object when retrieving it.
Conclusion
That’s it! Async Storage is a simple and effective way to store key value data locally in your React Native app. It’s perfect for saving things like user preferences, tokens, or any small amount of data that needs to persist across app sessions.
Now go ahead and try it out in your own project. With just a few lines of code, you can easily store and retrieve data on the user’s device.
Happy coding! 🎉
Leave a Reply